Automating Daily Email Reports with Python

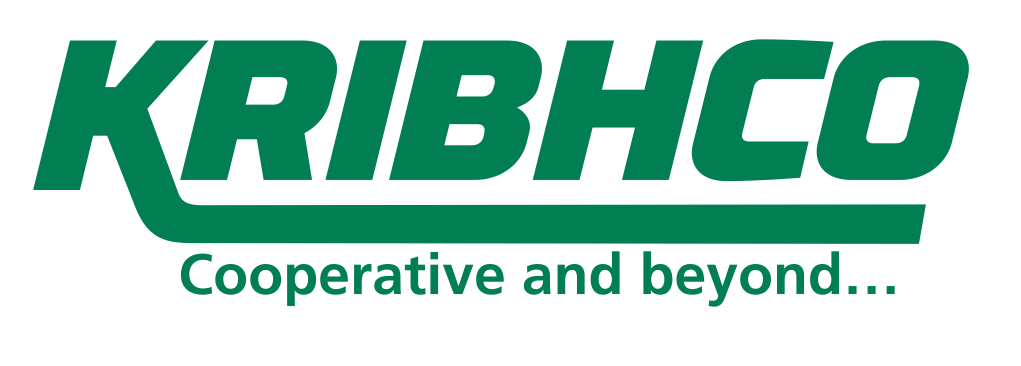
In today’s fast-paced world, automation is key to efficiency. One common task that many professionals face is sending daily email reports. Instead of manually sending these reports every day, why not automate the process? In this article, we’ll explore how to use Python to automate sending daily email reports.
The Python Script
Python, with its rich standard library, offers tools to send emails without the need for third-party libraries.
Here’s a simple script using the smtplib
and email
libraries:
import smtplib from email.message import EmailMessage def send_email(subject, content, to_email): EMAIL_ADDRESS = ‘your_email@gmail.com’ EMAIL_PASSWORD = ‘your_password’ msg = EmailMessage() msg.set_content(content) msg[‘Subject’] = subject msg[‘From’] = EMAIL_ADDRESS msg[‘To’] = to_email server = smtplib.SMTP(‘smtp.gmail.com’, 587) server.starttls() server.login(EMAIL_ADDRESS, EMAIL_PASSWORD) server.send_message(msg) server.quit() if __name__ == “__main__”: subject = “Daily Report” content = “Here is your daily report…” to_email = “recipient_email@example.com” send_email(subject, content, to_email) |
Setting It Up
- Gmail Configuration: The script assumes you’re using Gmail. For it to work, you need to enable less secure apps to access your Gmail account. It’s advisable to use a dedicated Gmail account for this purpose to maintain security.
- Script Customization: Modify the script by replacing placeholders with your Gmail credentials and the recipient’s email address.
- Automation: Depending on your operating system, you can automate the script to run daily:
- Windows: Use the Task Scheduler.
- Linux: Set up a
cron
job. - MacOS: Utilize
launchd
orcron
.
Security Considerations
- Password Storage: Storing your password directly in the script is a security risk. Instead, use environment variables or a secrets manager to keep your credentials safe.
- Less Secure Apps: Enabling access for less secure apps on your primary Gmail account is not recommended. Always use a secondary account for such tasks.
- Rate Limits: Gmail has sending rate limits. If you’re sending a high volume of emails, consider using dedicated email services like SendGrid or Mailgun.
Conclusion
Automating daily tasks like sending email reports can save time and reduce the chance of human error. With Python, this automation becomes straightforward and adaptable to various needs. Always remember to prioritize security when dealing with email automation to protect sensitive information.